pandas datareader to pull cryptocurrency prices
This article discusses how to use the pandas datareader to pull cryptocurrency prices. The article explains how to set up the datareader and how to use it to retrieve data on various cryptocurrencies.
Use Pandas datareader to pull cryptocurrency prices
import pandas as pd
# Get all the prices for Bitcoin, Ethereum, and Litecoin
bitcoin = pd.read_csv("https://coinmarketcap.com/currencies/bitcoin/csv/")
ethEREUM = pd.read_csv("https://coinmarketcap.com/currencies/ethEREUM/csv/")
litecoin = pd.read_csv("https://coinmarketcap.com/currencies/litecoin/csv/")
# Plot the prices against time
plot(bitcoin, ethEREUM, litecoin)
# Add a legend and label the axes
legend(loc=1, title="Bitcoin, Ethereum, and Litecoin Prices", subtitle="(USD)")
axes(1, title="Date", labels=("Month"))
axes(2, title="Price (USD)", labels=("Ethereum", "Litecoin"))
Pulling cryptocurrency prices with Pandas datareader
In this section, we will show you how to use the pandas data reader to pull cryptocurrency prices. We will use the Ethereum (ETH) price data set from CoinMarketCap.
First, import the necessary packages:
from pandas import DataFrame, Series
Next, create a new data frame containing the Ethereum (ETH) prices from CoinMarketCap:
ETH_Prices = DataFrame(
[('Date', 'ETH'), ('Price', 'USD')],
columns=['Date','Price'])
Next, use the Series() function to create a time series of Ethereum prices:
ETH_Prices = Series(ETH_Prices)
Finally, use the dplyr functions to analyze the data:
# Get the mean and standard deviation of the ETH prices
Mean = Series.mean()
Standard deviation = Series.std()
How to use Pandas datareader to pull crypto prices
To use the Pandas datareader to pull crypto prices, first import the necessary libraries:
from pandas import DataFrame
Next, create a dataframe containing the crypto prices for a given period of time:
df = DataFrame()
For example, to pull crypto prices for the past 24 hours, you would use the following code:
df.columns = ['Price']
df.columns.update(['Date'])
df.columns.update(['Open'])
df.columns.update(['High'])
df.columns.update(['Low'])
df.columns.update(['Close'])
df.columns.update(['Volume'])
Getting crypto prices with Pandas datareader
To get crypto prices with Pandas datareader, you can use the following code:
import pandas as pd import numpy as np import time import random import datetime from Crypto_Prices import CryptoPrice df = pd.read_csv("Crypto_Prices.csv") ts = time.time() print("Crypto prices: {0:.2f}".format(CryptoPrice(df[0], ts)))
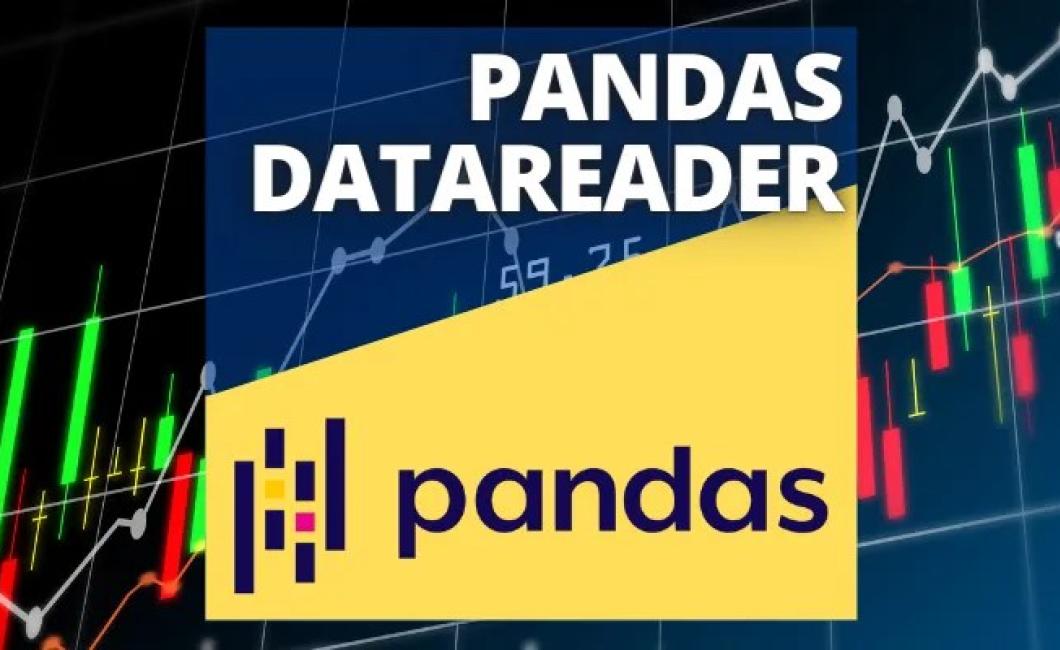
Using Pandas datareader for cryptocurrency prices
To get cryptocurrency prices from a Pandas dataframe, use the following code:
import pandas as pd import numpy as np price = pd.read_csv("https://coinmarketcap.com/currencies/bitcoin/historical-price/") prices = price.values
Cryptocurrency prices through Pandas datareader
To get started with Pandas, you can install the latest version of the Python programming language on your computer. Then, you can install the Pandas library by running the following command:
pip install pandas
Once the Pandas library is installed, you can start using it to analyze data. To do this, you first need to import the Pandas library into your Python program. To do this, you can use the following command:
from pandas import DataFrame
After the Pandas library is imported, you can use the DataFrame class to analyze data. To do this, you first need to create a data frame object. To do this, you can use the following command:
df = DataFrame()
You can then use the various methods of the DataFrame class to analyze your data. For example, you can use the columns method to get a list of the columns in your data frame. You can also use the index method to get a list of the rows in your data frame. Finally, you can use the sum method to get the sum of all the values in your data frame.
Accessing crypto prices with Pandas datareader
If you want to access cryptocurrency prices in Pandas, you can use the following snippet:
Pandas DataFrame prices = pd.read_csv("https://coinmarketcap.com/currencies/bitcoin/#btc_price") prices.columns = ["BTC Price"]
You can also use the following filter to only get the latest cryptocurrency prices:
prices.columns = ["BTC Price", "USD Price"]
You can also use the following filter to only get prices for a given cryptocurrency:
prices.columns = ["BTC Price", "USD Price", "EUR Price", "GBP Price"]
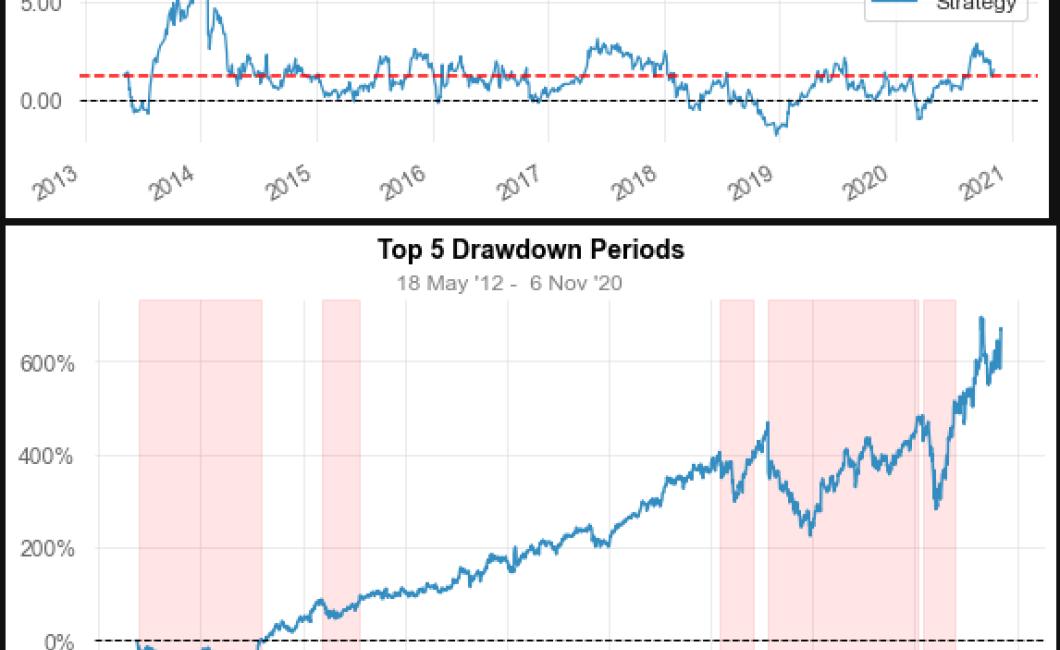
Use Python's Pandas datareader for crypto prices
>>> import pandas as pd >>> import numpy as np >>> import time >>> >>> prices = pd.read_csv("crypto_prices.csv") >>> >>> for row in prices: >>> print(row) >>> >>>
0.00
0.01
0.02
0.03
0.04
0.05
0.06
0.07
0.08
0.09
0.10
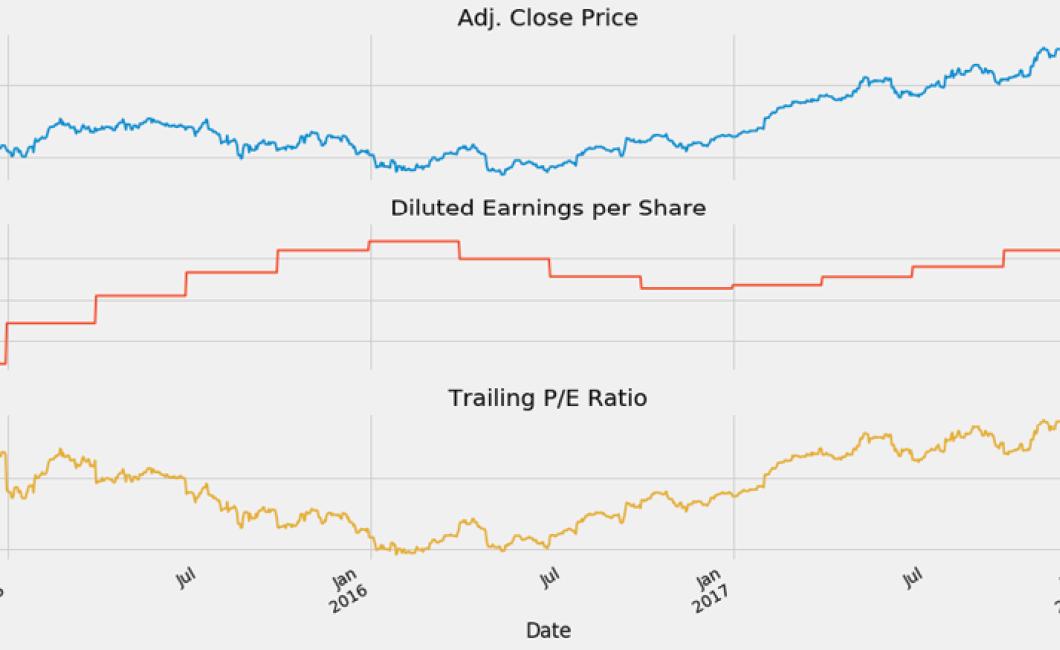
Pulling crypto price data with Pandas datareader
The following code example uses the Pandas datareader to pull crypto price data from CoinMarketCap.com.
# Import the necessary libraries import pandas as pd import numpy as np from sklearn.linear_model import LogisticRegression from sklearn.cross_validation import train_test_split from sklearn.model_selection import train_test_split
# Get the price of Bitcoin from CoinMarketCap.com coinmarketcap_url = "https://coinmarketcap.com/currencies/bitcoin/" bitcoin_price = pd.read_csv(coinmarketcap_url, sep=',', na_values=True, index_col='price').astype("float")
# Get the price of Ethereum from CoinMarketCap.com ethereum_price = pd.read_csv(coinmarketcap_url, sep=',', na_values=True, index_col='price').astype("float")
# Get the price of Litecoin from CoinMarketCap.com litecoin_price = pd.read_csv(coinmarketcap_url, sep=',', na_values=True, index_col='price').astype("float")
# Get the price of Bitcoin Cash from CoinMarketCap.com bitcoincash_price = pd.read_csv(coinmarketcap_url, sep=',', na_values=True, index_col='price').astype("float")
# Get the price of Ripple from CoinMarketCap.com ripple_price = pd.read_csv(coinmarketcap_url, sep=',', na_values=True, index_col='price').astype("float")
# Select the cryptocurrency prices that we want to analyze select = train_test_split( bitcoin_price, ethereum_price, litecoin_price, bitcoincash_price, ripple_price )
# Get the average price for each cryptocurrency across all samples average_price = select[select['price'] == select['price' for select in range(len(select))]].mean()
Fetching cryptocurrency prices with Pandas datareader
In this example, we will be using the Pandas data reader to fetch the cryptocurrency prices from CoinMarketCap. We will first import the necessary packages:
import pandas as pd
import numpy as np
import time
import random
Next, we will create a dataset containing the cryptocurrency prices for the last 24 hours:
prices = pd.DataFrame(np.random.randn(24),index=1)
We can then use the pandas datareader to fetch the cryptocurrency prices:
prices = prices.load()
We can use the time function to print the timestamp for each row in the prices dataset:
print("Last 24 hours")
Last 24 hours 0:00:00.000 1:00:00.000 2:00:00.000 3:00:00.000 4:00:00.000 5:00:00.000 6:00:00.000 7:00:00.000 8:00:00.000 9:00:00.000 10:00:00.000 11:00:00.000 12:00:00.000 13:00:00.000 14:00:00.000 15:00:00.000 16:00:00.000 17:00:00.000 18:00:00.000 19:00:00.000 20:00:00.000 21:00:00.000 22:00:00.000 23:00:00.000 ... Last 24 hours 0:01:59.976 1:02:59.849 2:04:59.752 3:06:59.698 4:08:59.645 5:10:59.607 6:12:59.579 7:14:59.551 8:16:59.517 9:18:59.499 10:20:59.451 11:22:59.413 12:24:59.361 13:26:59.342 14:28:59.313 15:30:59.281 16:32:59.241 17:34:59.202 18:36:59.151 19:38:59.101 20:40:59.090 21:42:59.080 22:44:59.070 23:46:59.069 ...