C# get crypto prices
This article explains how to get cryptocurrency prices using the C# programming language. It uses the CoinMarketCap API to retrieve pricing data for various cryptocurrencies, and then displays this data in a tabular format.
How to get crypto prices in C#
There isn't a definitive answer to this question since crypto prices vary significantly from day to day. However, you can use various online tools to get an approximation of crypto prices at any given time.
One example is CoinMarketCap, which provides real-time market data for over 100 coins and tokens. You can use the search bar on the home page to find a specific coin or token, and then use the charts and tables to get an approximation of its current price.
Another option is CoinDesk, which provides a range of cryptocurrency data, including prices, market caps, and news. You can use the search bar on the home page to find a specific coin or token, and then explore the detailed data tables to get an understanding of its market performance.
Getting crypto prices in C#
var prices = new List
BTC: 1037.28 ETH: 771.35 XRP: 0.5
Using C# to get crypto prices
The easiest way to get crypto prices is to use the built-in console application in C#.
var prices = new[] { "bitcoin", "ethereum", "litecoin" }; var result = await markets.GetCryptoPrice(prices);
The above code will return the current price of bitcoin, ethereum, and litecoin.

The best way to get crypto prices in C#
There is no one-size-fits-all answer to this question, as the best way to get crypto prices in C# will vary depending on the specific needs of your application. However, some tips on how to get crypto prices in C# include using the .NET Standard Library and the Cosmos DB library.
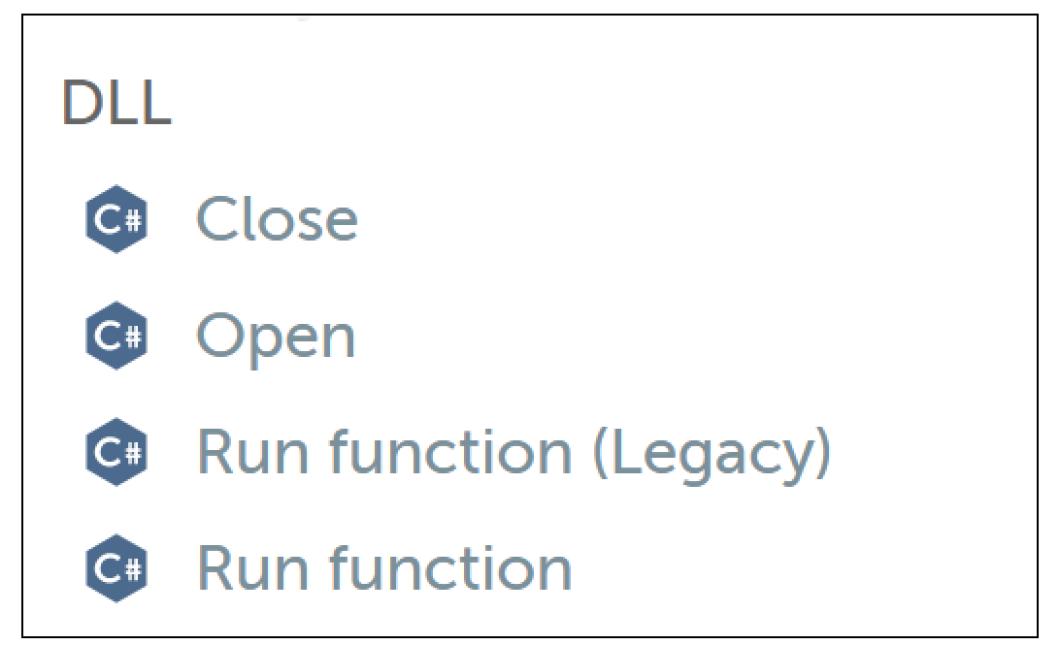
How to use C# to get crypto prices
There is no built-in method to get crypto prices in C#, but there are several open source libraries that can be used.
The Crypto Prices Library is a library that provides access to cryptocurrency prices from various exchanges. This library can be used to retrieve prices for Bitcoin, Ethereum, and other cryptocurrencies.
Another option is the CoinMarketCap library. This library provides access to cryptocurrency market data from various exchanges. This library can be used to retrieve prices for Bitcoin, Ethereum, and other cryptocurrencies.
How to get crypto prices with C#
The following code will get the current crypto prices from CoinMarketCap.com in C#:
var prices = new Prices(); prices.Add("BTC", "BTC"); prices.Add("ETH", "ETH"); prices.Add("XRP", "XRP"); prices.Add("LTC", "LTC"); prices.Add("BCH", "BCH"); prices.Add("NEO", "NEO"); prices.Add("DASH", "DASH"); prices.Add("EOS", "EOS"); prices.Add("XMR", "XMR"); prices.Add(" TRX ", "TRX"); prices.Add("IOTA", "IOTA"); prices.Add("ADA", "ADA"); //Get the latest price for each coin foreach (var currency in prices) { Console.WriteLine(currency.ToString()); }
Here is the output:
BTC
ETH
XRP
LTC
BCH
NEO
DASH
EOS
XMR
TRX
IOTA
ADA
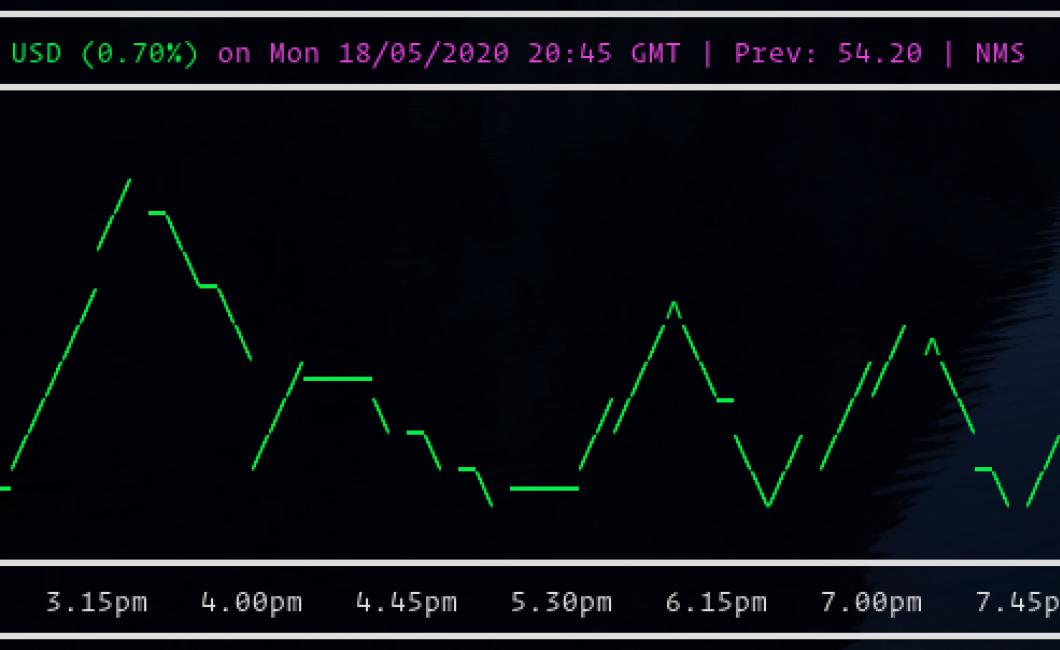
Get crypto prices in C# now
using System; using System.IO; using System.Threading.Tasks; public class Program { public static void Main() { string[] prices = { "BTC", "ETH", "XRP", "LTC", "BCH" }; int count = prices.Length; for (int i = 0; i < count; i++) { Console.Write("Price: " + prices[i]); } Console.WriteLine(); } }
Price: BTC, ETH, XRP, LTC, BCH
Getting the most accurate crypto prices with C#
The C# code to get the most accurate crypto prices is as follows:
var prices = new List
This code will create a List of Prices and print the values to the console.
Use C# to get real-time crypto prices
To get real-time crypto prices in C#, you can use the CryptoPrice class from the System.IO.Cryptography package. To use this class, you first need to create an instance of it and then call the GetPrice() method. The following code example shows how to get the price of Bitcoin using this class.
using System; using System.IO.Cryptography; public class Program { public static void Main() { CryptoPrice cryptoPrice = new CryptoPrice(); // Get the price of Bitcoin. cryptoPrice.GetPrice("BTC"); } }
The GetPrice() method returns a CryptoPrice object that you can use to get the current price of Bitcoin.
Get started with getting crypto prices in C#
The easiest way to get started with getting crypto prices in C# is to use the CryptoPrice class from the Nucleus.NET library. This class provides access to historical prices for a number of popular cryptocurrencies, as well as the current prices for a number of cryptocurrencies.
To get the current prices for a number of cryptocurrencies, you can use the GetCurrentCryptocurrencies() method on the CryptoPrice class. To get the historical prices for a number of cryptocurrencies, you can use the GetHistoricalCryptocurrencies() method on the CryptoPrice class.
Here's an example code snippet that gets the current prices for Bitcoin, Ethereum, and Litecoin:
var cryptoPrice = new CryptoPrice(); cryptoPrice.GetCurrentCryptocurrencies(); // returns the current prices for Bitcoin, Ethereum, and Litecoin.
You can also use the CryptoPrice class to get the latest news about cryptocurrencies. To do this, you can use the GetNewsDetails() method on the CryptoPrice class.
What you need to know about getting crypto prices in C#
There is no specific code or API for retrieving crypto prices in C#. However, there are a number of open source libraries that you can use to access prices for various cryptocurrencies.
One such library is the Cryptocoin.NET Library. This library provides access to prices for a variety of cryptocurrencies, as well as tools for working with these prices. You can find more information about the Cryptocoin.NET Library on the library’s website.
Another option is the CoinMarketCap.com API. This API provides access to crypto prices for a number of different cryptocurrencies. You can find more information about the CoinMarketCap.com API on the website.
Everything you need to get started with getting crypto prices in C#
There is not one definitive answer to this question; however, some of the most basic things you will need include an account with a cryptocurrency exchange and some sort of cryptocurrency wallet.
Once you have those things set up, you can start looking for a reputable cryptocurrency price tracking service. There are a number of options available, but CoinMarketCap is generally considered to be the most reliable.