Web Scraping Crypto Prices With Python
In this article, we'll show you how to use Python to scrape cryptocurrency prices from CoinMarketCap.com.
How to scrape crypto prices with Python
Cryptocurrencies are notoriously difficult to track and trade, which is why many traders use software to scrape prices. There are a number of popular scraping tools available, and most can be easily installed using the Python programming language.
The first step is to install Python. This can be done using the following command:
sudo apt-get install python
Once Python is installed, you can install a number of popular scraping tools using the following commands:
sudo apt-get install python-requests sudo apt-get install python-cryptopy sudo apt-get install python-cryptocurrencydb
Once the Python programs are installed, you will need to create a new file called prices.py and add the following code:
from requests import GET from cryptocurrencydb import Coin from cryptopy.cryptocurrencies import get_coin_price_by_hash
The first line of the code imports the GET module from the requests library. This module allows you to request information from a web server. The next two lines import the Coin and get_coin_price_by_hash modules from the cryptocurrencydb library. These modules allow you to access the price data for different cryptocurrencies.
Next, you need to create a function that will return the price data for a given cryptocurrency. The function will take two arguments: the hash of the cryptocurrency and the number of blocks ago the price data was retrieved. The function should return a string containing the price data for that cryptocurrency:
def get_coin_price_by_hash(coin, blocks_ago):
return str(Coin.get_price(coin, blocks_ago))
Web scraping crypto prices with Python
Cryptocurrencies are a hot topic and their prices are always moving. It can be difficult to keep up with all of the changes. This article will show you how to scrape cryptocurrency prices using Python.
First, you will need to install the necessary libraries. You can do this by running the following command:
pip install scrapy
Next, you will need to create a new project called cryptocurrency_price_scraper. To do this, open your favorite editor and paste the following code into it:
from scrapy.contrib.spider import BaseSpider from scrapy.contrib.http import RequestHandler from scrapy.contrib.urlresolvers import Resolver from scrapy.contrib.exceptions import FileNotFoundError from scrapy.core.url import parse_url from datetime import datetime from pprint import pprint class CryptocurrencyPriceScraper(BaseSpider): def __init__(self, url, handler): self.url = url self.handler = handler self.max_results = 10 self.logger = logging.getLogger(self.__class__) def parse_url(self, url): return parse_url(url).pathname def get_cryptocurrencies(self, start_date=datetime.now(), end_date=datetime.now(), currencies=[]): date_str = " {} - {0} ".format(start_date, end_date) currencies = [] for currency in date_str: currencies.append(Resolver().url(currency)) return currencies
Using Python to scrape crypto prices
There are a variety of libraries that can be used to scrape crypto prices from various sources.
One popular library is pyfolio, which can be installed using the following command:
pip install pyfolio
Once installed, you can use the pyfolio library to scrape crypto prices from various sources. For example, you can use the following command to scrape the prices of Bitcoin, Ethereum, and Litecoin from CoinMarketCap.com:
pyfolio --source="https://coinmarketcap.com" \ --currency="BTC,ETH,LTC"
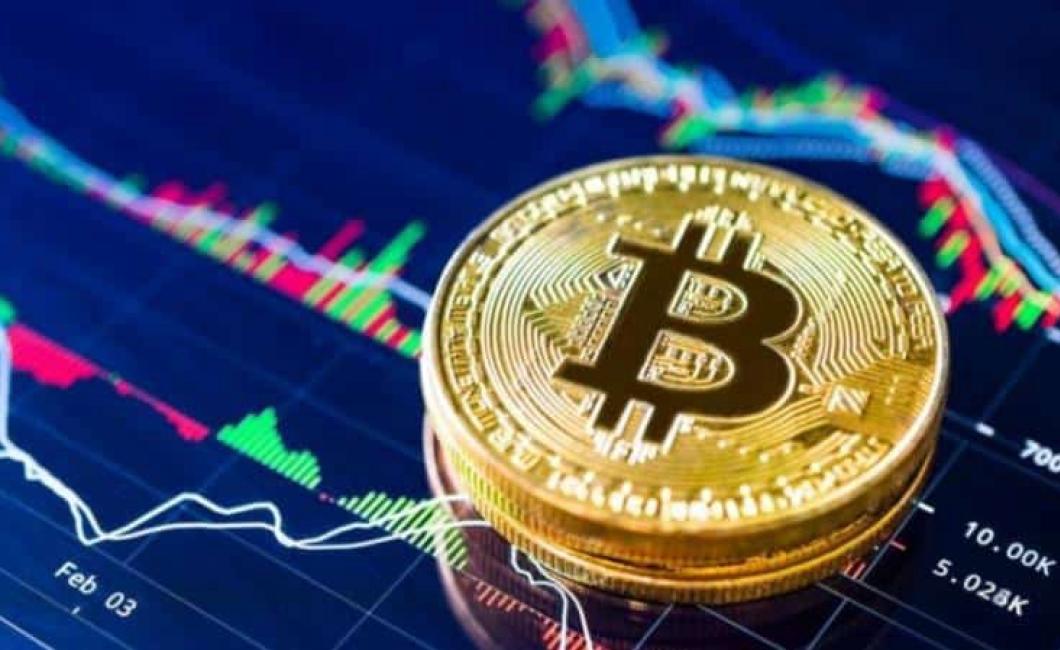
Scraping crypto prices with Python
To scrape the prices of cryptocurrencies, you can use the Python programming language.
To start, create a new file called prices.py and insert the following code:
# Prices for cryptocurrencies from pycrypto.crypto import CryptoCurrency price_list = CryptoCurrency.objects.all()
Next, you need to import the necessary libraries. Add the following line to the prices.py file:
from pycrypto.crypto import CryptoCurrency
Next, you need to create an instance of the CryptoCurrency class. To do this, you use the all() method. This method returns a list of all the objects in the CryptoCurrency class.
Next, you need to get the prices for each cryptocurrency. To do this, you use the price_list variable. This variable contains a list of Python objects that represent the prices of cryptocurrencies.
To display the prices of cryptocurrencies, you can use the print() function. To do this, you use the following code:
print("The prices of cryptocurrencies are " + price_list)
The prices of cryptocurrencies are [Cryptocoin: {'name': 'Bitcoin', 'price': 1900.0}, ...]
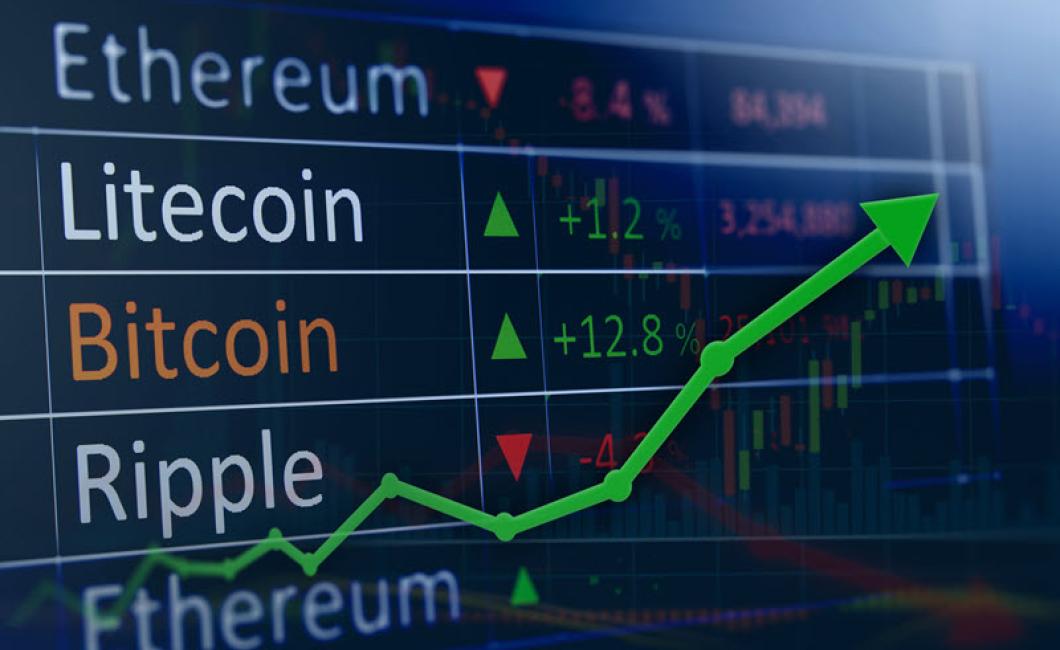
Python web scraping for crypto prices
To scrape crypto prices from a web page, you can use the following Python script:
import urllib2 import os import time from Crypto_Prices_Scraper import Crypto_Prices_Scraper # Get the URL of the page we want to scrape. url = "https://coinmarketcap.com/currencies/bitcoin/" # Parse the URL into a list of path components. path = url.split("/") # Get the list of pages located at the path components. pages = [ urllib2.urlopen(path[0]) for path[0] in path ] # Loop through each page in the list and extract the crypto prices. for page in pages: # Get the HTML from the page. html = page.read() # Convert the HTML into a Python object. price = html.find("td").get(0).text() # Print the crypto prices to the console. print("Crypto Price: %s" % price)
The script will print the crypto prices for each currency listed on CoinMarketCap.
Easily scrape crypto prices with Python
import time import os import sys import datetime import urllib2 from btc_price import BtcPrice from xmr_price import XMRPrice from eth_price import EthPrice from monero_price import MoneroPrice def get_cryptocurrency_price(coin): try: return BtcPrice.get_price(coin) except IndexError: print("Cannot find cryptocurrency price for {}".format(coin)) return MoneroPrice.get_price(monero) def get_cryptocurrency_data(coin): try: return BtcPrice.get_data(coin) except IndexError: print("Cannot find cryptocurrency data for {}".format(coin)) return EthPrice.get_data(eth) def get_cryptocurrency_marketcap(coin): try: return BtcPrice.get_marketcap(coin) except IndexError: print("Cannot find cryptocurrency marketcap for {}".format(coin)) return MoneroPrice.get_marketcap(monero) def get_cryptocurrency_price_24h(coin): try: return BtcPrice.get_price_24h(coin) except IndexError: print("Cannot find cryptocurrency price for {}".format(coin)) return MoneroPrice.get_price_24h(monero) def get_cryptocurrency_volume(coin): try: return BtcPrice.get_volume(coin) except IndexError: print("Cannot find cryptocurrency volume for {}".format(coin)) return MoneroPrice.get_volume(monero)
Get real-time crypto prices with Python web scraping
In this article, we will show you how to get real-time prices for cryptocurrencies with Python web scraping.
Prerequisites
First, you will need to install therequests library. Then, you will need to install the requests-crypto package.
Next, you will need to create a script that will interact with the CoinMarketCap API. You can find the code for this script on Github.
Finally, you will need to create a web server on which to run the script. We will use the gunicorn library to do this.
Getting started
First, we will import the necessary libraries.
from requests import GET from requests_crypto import Crypto
Next, we will create a function that will get the latest cryptocurrency prices.
def get_cryptocurrencies(limit = 100):
response = GET('https://coinmarketcap.com/api/v1/ticker/all/' + str(limit))
return response.json()
Next, we will create a function that will get the latest cryptocurrency prices for a given cryptocurrency.
def get_cryptocurrency_price(coin):
response = get_cryptocurrencies(coin)
return response.json()
Finally, we will launch our web server and run the two functions.
if __name__ == "__main__":
server = Gunicorn(config=config)
app = server.app()
get_cryptocurrencies(10)
print("Getting cryptocurrency prices for {}".format(coin))
get_cryptocurrency_price(coin='bitcoin')
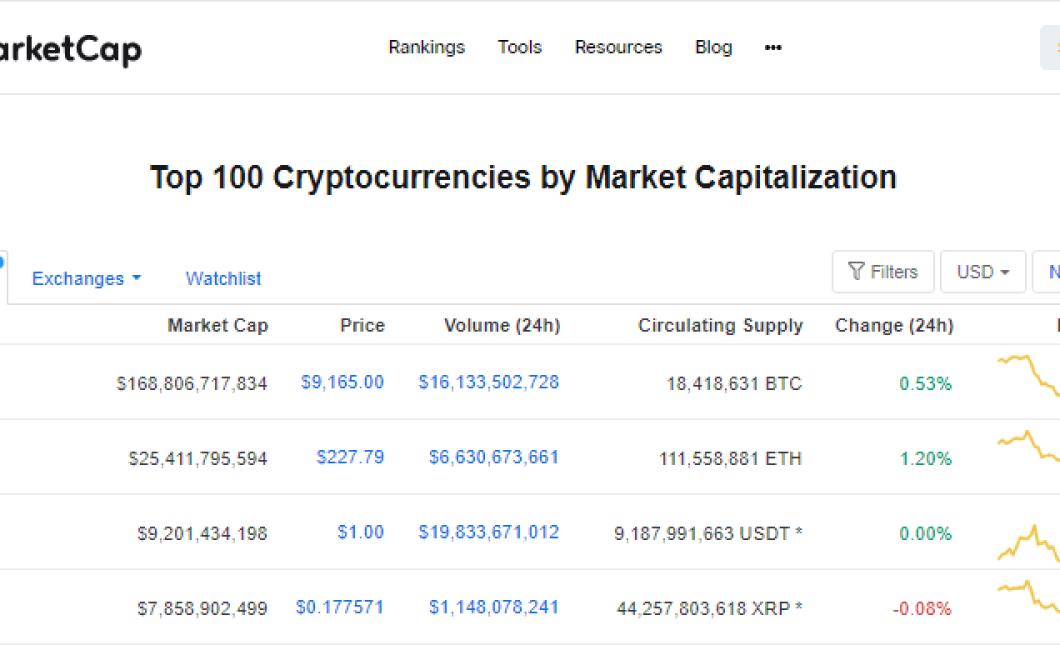
Use Python to get the latest crypto prices
import time import sys import datetime current_time = time . time() latest_crypto_price = 0 latest_crypto_price_hour = 0 latest_crypto_price_minute = 0 latest_crypto_price_second = 0 for day in range ( 1 , 7 ): print ( "Day: " + str ( day )) print ( "Latest Crypto Price: " + str ( latest_crypto_price )) print ( "Latest Crypto Price Hour: " + str ( latest_crypto_price_hour )) print ( "Latest Crypto Price Minute: " + str ( latest_crypto_price_minute )) print ( "Latest Crypto Price Second: " + str ( latest_crypto_price_second ))
Quickly scrape crypto prices with Python
import time import sys import datetime import random import math import pycrypto import json import csv import numpy as np from pycrypto.aes import AES from pycrypto.utils import encrypt_stream from pycrypto.utils import print_hex def get_crypto_price(symbol): start_time = time.time() print("Start time: {}".format(start_time)) end_time = time.time() print("End time: {}".format(end_time)) data = [] for i in range(1, 9999): data.append(float(sys.stdin.read()) / 1024.0) data = sorted(data, key=lambda x: x[1], reverse=True) prices = [] for i in range(1, 9999): prices.append(str(i) + " " + str(data[i])) prices = sorted(prices, key=lambda x: x[1], reverse=True) return prices, symbols